SwiftUI での AdMob 実装に少し手こずったので備忘録。
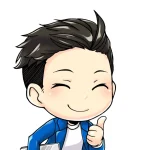
同じように手こずってる人は、ぜひ参考にしてみてください。
- Xcode 13.2
- Swift 5.5
CocoaPods で Mobile Ads SDK をインポート
SDK のインポートには CocoaPods を使用。プロジェクトの Podfile を開いてアプリのターゲットに以下を追加します。
pod 'Google-Mobile-Ads-SDK'
ターミナルで以下のコマンドを実行 。
pod install --repo-update
Info.plist を更新
Info.plist にキーを 2 つ追加します。
GADApplicationIdentifier キーの追加
ca-app-pub-xxxxxxxxxxxxxxxxx~xxxxxxxxxxx
の部分には、あなたのアプリIDを入れます。(こちらから確認できます )
<key>GADApplicationIdentifier</key>
<string>ca-app-pub-xxxxxxxxxxxxxxxxx~xxxxxxxxxxx</string>
SKAdNetworkItems キーの追加
<key>SKAdNetworkItems</key>
<array>
<dict>
<key>SKAdNetworkIdentifier</key>
<string>cstr6suwn9.skadnetwork</string>
</dict>
<dict>
<key>SKAdNetworkIdentifier</key>
………
上のものは情報が古い可能性があるので、最新の内容を以下のリンクからコピペしてください。
Xcode で Mobile Ads SDK を初期化
まずは GoogleMobileAds
をインポートします。
import SwiftUI
import GoogleMobileAds
初期化するタイミングとしてはapplication(_:didFinishLaunchingWithOptions:)
が解りやすいと思うので、UIApplicationDelegate
に準拠したクラスを定義します。
import SwiftUI
import GoogleMobileAds
class AppDelegate: NSObject, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions
launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
}
}
@main
struct DemoApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
application(_:didFinishLaunchingWithOptions:)
内で Mobile Ads SDK を初期化します。
import SwiftUI
import GoogleMobileAds
class AppDelegate: NSObject, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions
launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
GADMobileAds.sharedInstance().start(completionHandler: nil) //Mobile Ads SDK の初期化
return true
}
}
@main
struct DemoApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
SwiftUI でも AppDelegate クラスの作成と管理がおこなえるよう@UIApplicationDelegateAdaptor
を使用します。
import SwiftUI
import GoogleMobileAds
class AppDelegate: NSObject, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions
launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
GADMobileAds.sharedInstance().start(completionHandler: nil) //Mobile Ads SDK の初期化
return true
}
}
@main
struct DemoApp: App {
@UIApplicationDelegateAdaptor(AppDelegate.self) var appDelegate
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
バナー広告の実装
バナー広告表示用のビューを作成します。(ここではAdMobBannerView
)
UIKit を SwiftUI アプリで取り扱うためUIViewRepresentable
に準拠させています。
struct AdMobBannerView: UIViewRepresentable {
func makeUIView(context: Context) -> GADBannerView {
let bannerView = GADBannerView(adSize: GADAdSizeBanner)
}
func updateUIView(_ uiView: GADBannerView, context: Context) {
}
}
GADBannerView のプロパティ(adUnitID
・rootViewController
)を設定します。
struct AdMobBannerView: UIViewRepresentable {
func makeUIView(context: Context) -> GADBannerView {
let bannerView = GADBannerView(adSize: GADAdSizeBanner)
//adUnitID は広告を読み込む先の広告ユニットのID。です。(アプリ配信時には自分の広告ユニットIDに置き換える)
bannerView.adUnitID = "ca-app-pub-3940256099942544/2934735716" //バナー広告テスト用の広告ユニットID
let windowScenes = UIApplication.shared.connectedScenes.first as? UIWindowScene
bannerView.rootViewController = windowScenes?.keyWindow?.rootViewController
}
func updateUIView(_ uiView: GADBannerView, context: Context) {
}
}
loadRequest:
で広告をリクエストし、ビューを返します。
struct AdMobBannerView: UIViewRepresentable {
func makeUIView(context: Context) -> GADBannerView {
let bannerView = GADBannerView(adSize: GADAdSizeBanner)
//バナー広告テスト用の広告ユニットIDです。配信時はご自身の広告ユニットIDに置き換えてください。
bannerView.adUnitID = "ca-app-pub-3940256099942544/2934735716"
let windowScenes = UIApplication.shared.connectedScenes.first as? UIWindowScene
bannerView.rootViewController = windowScenes?.keyWindow?.rootViewController
bannerView.load(GADRequest())
return bannerView
}
func updateUIView(_ uiView: GADBannerView, context: Context) {
}
}
バナー広告の呼び出し
以下のコードで好きな場所にバナー広告を配置できます。
AdMobBannerView()